Understanding the distinctions between Middleware, Guards, and Interceptors in NestJS is essential for writing clean, scalable, and maintainable applications. Although they might seem similar at first glance, each of these components has a unique role in the request-response lifecycle.
In this post, we’ll explore the differences, use cases, and when to use what in your NestJS application.
What is Middleware in NestJS?
Middleware functions are called before the request reaches the route handler. Think of them as the “gatekeepers” of HTTP requests. Middleware can modify the request and response objects and decide whether or not the request should continue through the pipeline.
Common Use Cases for Middleware
- Logging incoming requests
- Request validation or sanitisation
- Authentication token parsing
- Applying CORS or security headers
Example
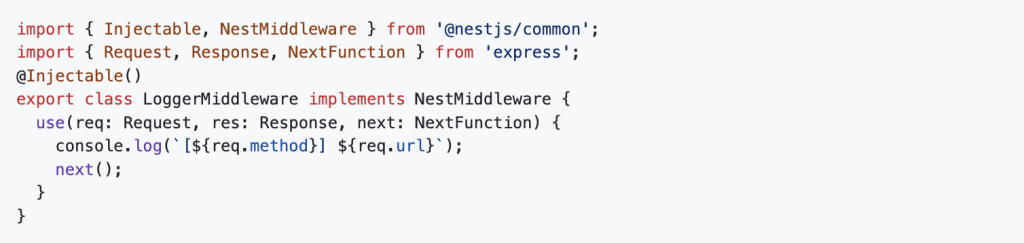
This middleware logs every incoming request. Middleware is typically applied in main.ts
or a module file using configure()
method.
What are Guards in NestJS?
Guards come after Middleware in the lifecycle and are used for authorization and access control. They determine whether a user can proceed to a specific route or not.
Guards return true
or false
(or throw exceptions) and are often used with roles and permissions.
Common Use Cases for Guards
- Role-based access control
- Route protection
- JWT verification
- Multi-tenant checks
Example
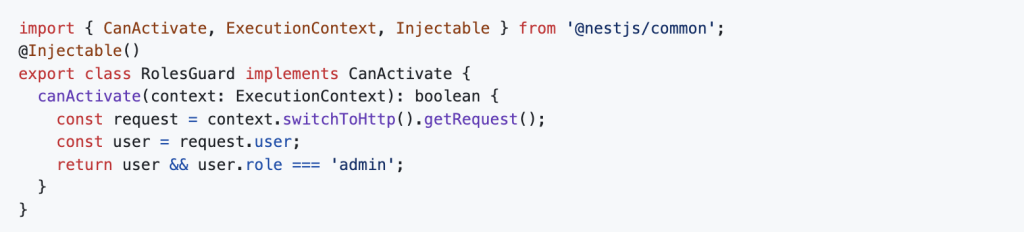
Apply it using @UseGuards(RolesGuard)
on routes or controllers.
What are Interceptors in NestJS?
Interceptors sit after guards and are used for manipulating the request or response, logging, transformation, or even retry logic. They wrap around the method execution and can be used to transform the result, measure performance, or implement caching.
Common Use Cases for Interceptors
- Transforming responses (e.g., exclude sensitive data)
- Logging and metrics
- Error formatting
- Timeout and retry logic
Example
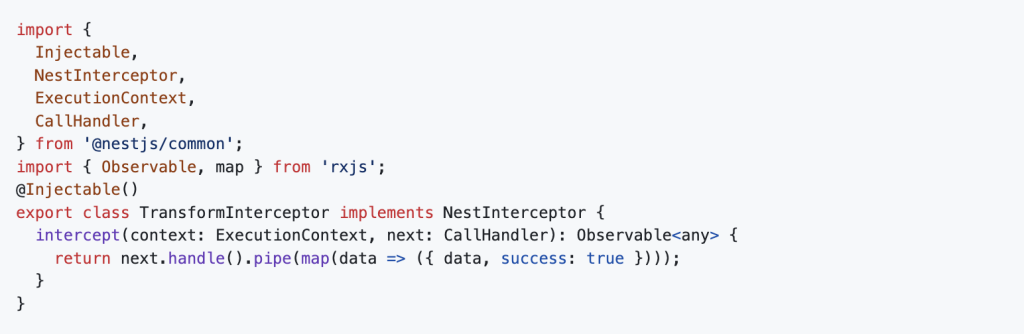
This interceptor wraps the response in a consistent format.
Lifecycle Order: Middleware → Guard → Interceptor → Controller
To clarify the flow:
- Middleware runs first — modifies the request object if needed.
- Guards evaluate permissions and access.
- Interceptors intercept and optionally modify the method execution or its result.
- Controller handles the final logic of the request.
Understanding this flow is crucial for placing logic in the right place.
When to Use What?
Feature | Purpose | Runs When | Best For |
---|---|---|---|
Middleware | Pre-process request | Before reaching controllers | Logging, parsing, headers |
Guards | Authorisation | Before route execution | Permissions, roles, access logic |
Interceptors | Transform, log, handle responses | Before & after method execution | Response shaping, error handling |
Each component serves a distinct purpose. Use middleware for broad concerns, guards for access control, and interceptors for response manipulation or performance logging. |
Final Thoughts
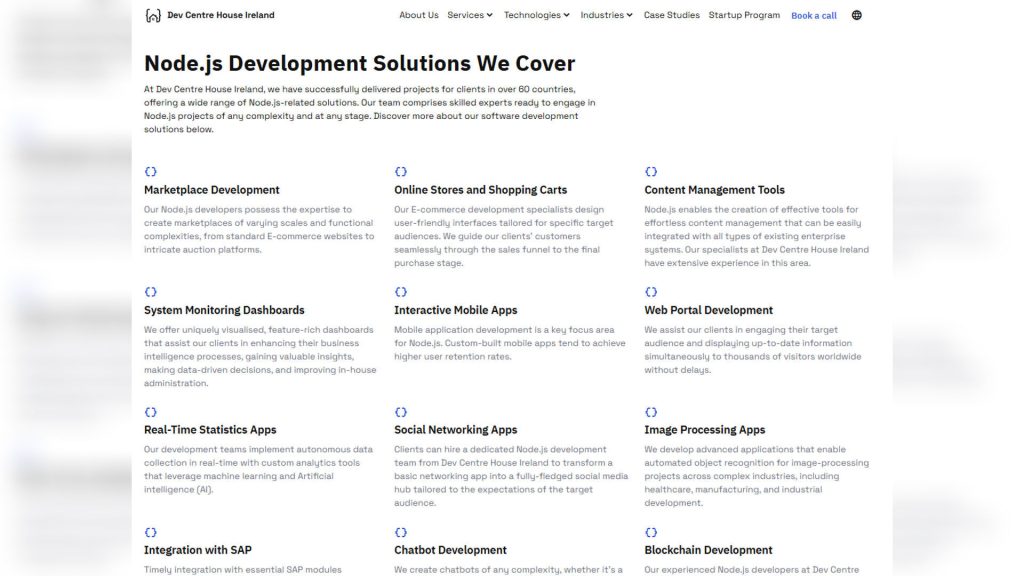
Knowing the difference between NestJS Middleware vs Guards vs Interceptors is key to writing clean and efficient backend logic. Use them strategically to build secure, well-structured applications that are easy to maintain and scale.
To further enhance your NestJS backend, check out how professional teams build Node.js backends at Dev Centre House Ireland.