Node.js is widely used for building scalable APIs, but performance bottlenecks can hinder its efficiency. Optimising your API ensures fast response times, reduced latency, and a better user experience. Here are ten proven techniques to enhance your Node.js API performance.
1. Enable Gzip Compression
Compressing responses reduces payload size, improving load times. Use the compression
middleware in Express to enable Gzip:

2. Use Asynchronous Code Efficiently
Blocking operations can slow down your API. Use asynchronous programming with async/await
or Promises to handle I/O tasks efficiently:

3. Optimise Database Queries
Inefficient queries can degrade API performance. Use indexing, pagination, and query optimisations to improve database efficiency. For example, in MongoDB:

4. Implement Caching
Caching prevents redundant computations and database queries. Use Redis to cache frequently requested data:
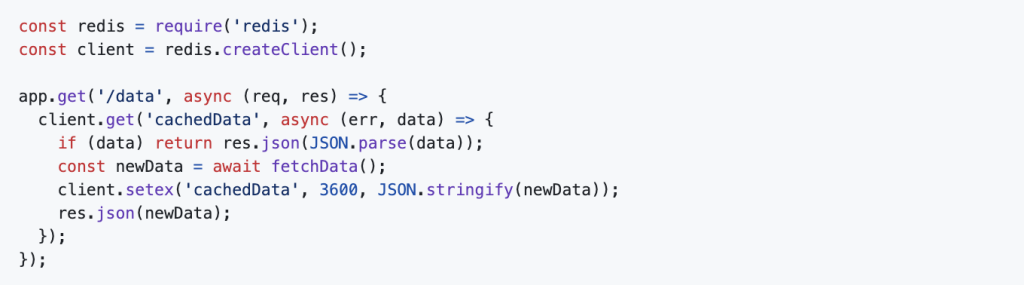
5. Use Cluster Mode
Node.js runs on a single thread by default. Utilising clustering can take advantage of multi-core processors:
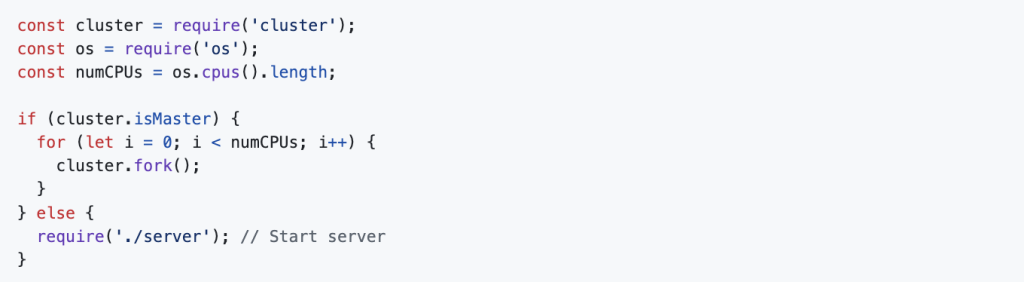
6. Reduce Middleware Overhead
Too many middlewares can slow down requests. Use only necessary middleware and avoid redundant processing.
7. Use Connection Pooling
For APIs with high database traffic, connection pooling improves efficiency. In PostgreSQL, use pg-pool
:

8. Limit Payload Size
Restrict request sizes to prevent excessive processing. In Express, set limits in body-parser
:

9. Implement Rate Limiting
Prevent abuse and API overuse by applying rate limiting using express-rate-limit
:

10. Monitor and Profile Performance
Use tools like pm2
, New Relic
, or Node.js Performance Hooks
to monitor your API and identify bottlenecks.
By implementing these techniques, you can significantly improve the speed and efficiency of your Node.js API, leading to better scalability and performance. Try them out and experience the difference!
Node.js: Key Takeaways from Dev Centre House Ireland
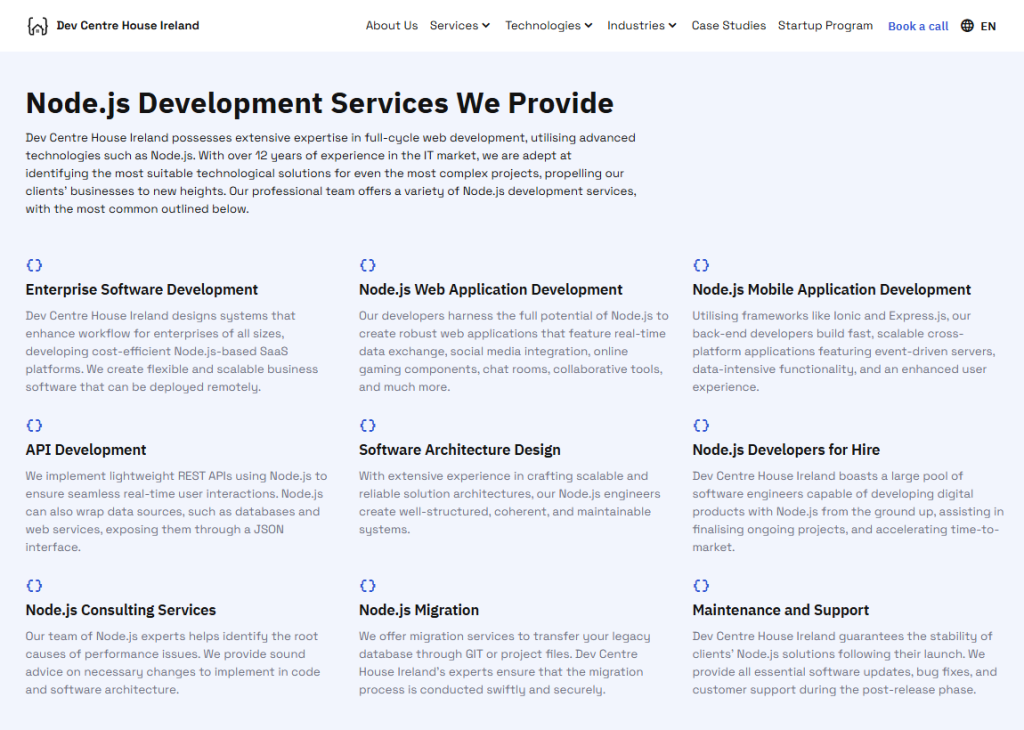
In conclusion, Node.js stands out as a powerful and versatile runtime environment for building scalable and high-performance backend applications. Its event-driven, non-blocking architecture, coupled with the vast npm ecosystem, enables developers to create efficient and responsive solutions. Whether you’re building real-time applications, APIs, or microservices, Node.js offers a robust foundation for modern web development, making it a valuable asset in any developer’s toolkit.
For further exploration and learning, refer to the detailed information provided in the resource: https://www.devcentrehouse.eu/en/technologies/back-end/nodejs.