Microservices architecture has become a go-to approach for building scalable and maintainable applications, and Node.js is one of the most popular frameworks for developing them. With its event-driven, non-blocking I/O model, Node.js enables high-performance microservices that can efficiently handle concurrent requests.
However, building scalable microservices in Node.js requires best practices to ensure performance, reliability, and maintainability.
In this guide, we’ll cover eight critical tips to help you design efficient, robust, and future-proof microservices in Node.js.
1. Use a Monorepo for Better Code Management
Managing multiple microservices can become a challenge as your system grows. Instead of handling separate repositories for each service, consider using a monorepo structure.
Why?
- Easier dependency management – Share packages across services.
- Consistent versioning – Avoid compatibility issues between services.
- Simplified CI/CD pipelines – Deploy all microservices efficiently.
Tool Suggestion: Use Nx or Lerna for managing monorepos in a scalable way.
2. Implement API Gateway for Centralized Routing
Microservices often expose multiple APIs, making it complex for clients to interact with them. A centralized API gateway helps streamline communication and manage authentication, caching, rate limiting, and logging in one place.
Why?
- Reduces complexity – Clients interact with a single API.
- Improves security – Apply authentication and authorization at one entry point.
- Optimizes performance – Use caching and load balancing.
Tool Suggestion: Use Kong, NGINX, or Express Gateway for API management.
3. Use Asynchronous Communication with Message Queues
While REST APIs are useful, microservices scale better with asynchronous communication using message queues. This prevents bottlenecks and ensures high availability.
Why?
- Decouples microservices – Services don’t wait for responses.
- Handles high loads – Queues prevent request overload.
- Improves fault tolerance – Failed messages can be retried.
Tool Suggestion: Use RabbitMQ, Apache Kafka, or NATS for event-driven communication.
4. Containerize Microservices with Docker
Deploying microservices individually can be painful. Using Docker containers simplifies the process by ensuring that each microservice runs with the same environment across different machines.
Why?
- Portable and lightweight – Run anywhere without compatibility issues.
- Easier scaling – Deploy multiple instances quickly.
- Better dependency management – No more “it works on my machine” issues.
Tool Suggestion: Use Docker Compose for local development and Kubernetes for production orchestration.
5. Implement Proper Logging and Monitoring
Microservices generate large volumes of logs. Without structured logging and monitoring, debugging becomes difficult.
Best Practices:
- Use a centralized logging system (e.g., ELK Stack, Loki, or Fluentd).
- Include correlation IDs to track requests across services.
- Set up real-time monitoring using Prometheus and Grafana.
Tool Suggestion: Use Winston or Pino for logging in Node.js.
6. Secure Microservices with Authentication and Authorization
Security is critical in microservices. Implement robust authentication and authorization mechanisms to prevent unauthorized access.
Best Practices:
- Use OAuth 2.0 and OpenID Connect for secure authentication.
- Implement JWT (JSON Web Tokens) for stateless authentication.
- Restrict access using Role-Based Access Control (RBAC) or Attribute-Based Access Control (ABAC).
Tool Suggestion: Use Keycloak, Auth0, or Passport.js for authentication.
7. Optimize Database Access with Connection Pooling
Database bottlenecks can significantly impact the scalability of microservices. Each service should efficiently manage database connections.
Best Practices:
- Use connection pooling to reuse database connections.
- Avoid long-running transactions that lock resources.
- Implement database replication and sharding for better performance.
Tool Suggestion: Use pg-pool for PostgreSQL or Sequelize with pooling options in Node.js.
8. Automate Deployment with CI/CD Pipelines
Manual deployments can introduce errors and delays. Automated CI/CD pipelines ensure that code changes are tested and deployed seamlessly.
Best Practices:
- Use GitHub Actions, GitLab CI/CD, or Jenkins for automation.
- Implement blue-green deployments to minimize downtime.
- Use feature flags for controlled rollouts.
Tool Suggestion: Use ArgoCD or Spinnaker for Kubernetes-based deployments.
Final Thoughts: Build Scalable Node.js Microservices the Right Way
Scalability and maintainability are the core principles of microservices architecture. By implementing these best practices, you ensure that your Node.js microservices remain highly performant, secure, and resilient.
Quick Recap of Best Practices:
Best Practice | Benefit |
---|---|
Monorepo | Centralized code management |
API Gateway | Simplifies routing & security |
Message Queues | Asynchronous, scalable communication |
Docker & Kubernetes | Portable, containerized deployment |
Logging & Monitoring | Easy debugging & observability |
Authentication & Security | Protects microservices from threats |
Database Connection Pooling | Improves performance |
CI/CD Automation | Faster, error-free deployments |
By applying these critical tips, you’ll be able to develop robust, future-proof microservices in Node.js that can handle large-scale applications efficiently. |
Concluding Insights: Node.js, via Dev Centre House Ireland
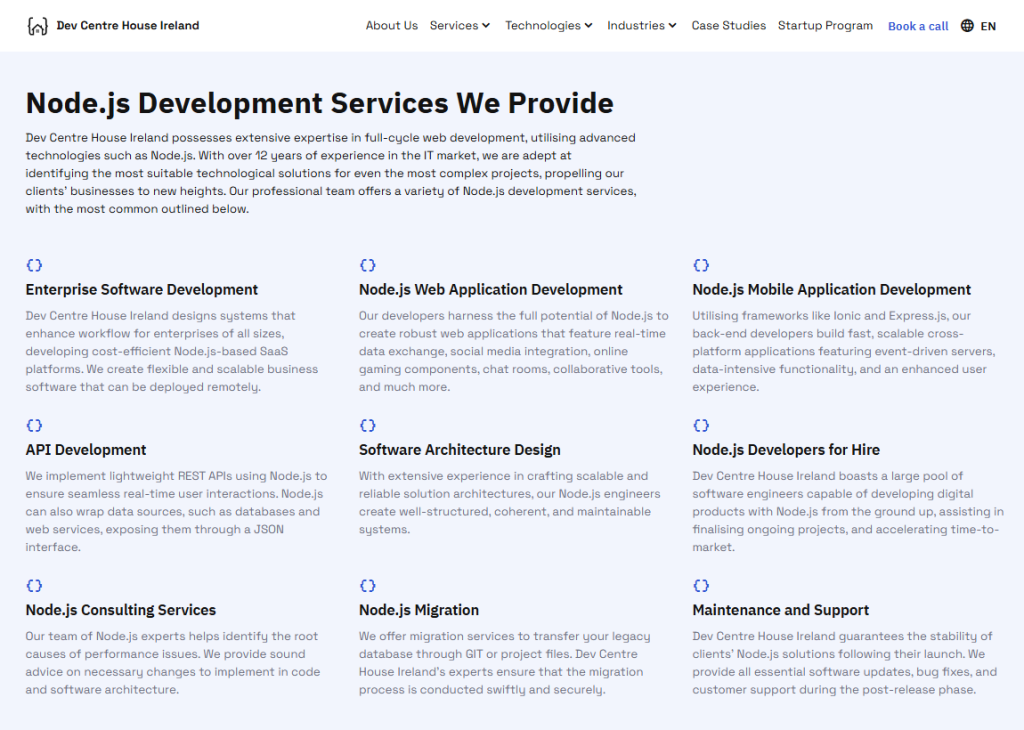
In conclusion, Node.js stands out as a powerful and versatile runtime environment for building scalable and high-performance backend applications. Its event-driven, non-blocking architecture, coupled with the vast npm ecosystem, enables developers to create efficient and responsive solutions. Whether you’re building real-time applications, APIs, or microservices, Node.js offers a robust foundation for modern web development, making it a valuable asset in any developer’s toolkit.
For further exploration and learning, refer to the detailed information provided in the resource: https://www.devcentrehouse.eu/en/technologies/back-end/nodejs.