In the world of modern web development, performance and scalability are everything. Whether you’re handling a few thousand concurrent users or building real-time applications that respond instantly, one feature in Node.js quietly powers it all: the Node.js Event Loop. Understanding how this mechanism works can help developers write more efficient code, troubleshoot bottlenecks, and build blazing-fast applications.
This article explores the Node.js Event Loop, how it works behind the scenes, and why it’s vital for maintaining high performance in Node.js applications. We’ll also look at how this architecture differs from traditional models and why developers around the globe trust Node.js for real-time, non-blocking workloads.
What is the Event Loop in Node.js?
At its core, Node.js is a single-threaded, event-driven runtime environment. Unlike traditional multi-threaded servers, Node.js uses a non-blocking I/O model, which is made possible by the Event Loop. This design allows Node.js to handle multiple operations simultaneously despite operating on a single thread.
The Event Loop acts as the manager of all asynchronous tasks. When operations such as file reads, network calls, or timers are triggered, they don’t block the main thread. Instead, they’re handled in the background, and the Event Loop decides when to run the callback associated with each task.
Why Is the Event Loop Crucial for Performance?
Many programming environments use multithreading to handle concurrency. But threads can be resource-heavy and complex to manage. Node.js simplifies this by offloading I/O to the system kernel and using the Event Loop to handle execution.
Here’s why the Event Loop enhances Node.js performance:
- Non-blocking nature: Tasks like HTTP requests or database queries don’t pause the execution of code.
- High scalability: Node.js can manage thousands of connections with minimal overhead.
- Efficient memory usage: Single-threaded architecture avoids the cost of managing multiple threads.
For real-time applications such as chat systems, gaming platforms, or live dashboards this performance model is ideal.
How the Event Loop Works: Step-by-Step
The Event Loop follows a specific sequence of phases that run continuously as long as there are callbacks to execute. These phases include:
1. Timers
This phase executes callbacks scheduled by setTimeout()
and setInterval()
.
2. Pending Callbacks
Executes I/O callbacks that were deferred to the next loop iteration.
3. Idle, Prepare
Internal use only. Not commonly used by developers.
4. Poll
Retrieves new I/O events. If there are no timers or I/O events, it waits for callbacks.
5. Check
This is where setImmediate()
callbacks are executed.
6. Close Callbacks
Handles close
events, e.g., socket.on('close')
.
In between these phases, Node.js also checks the microtask queue (e.g., process.nextTick()
or Promises), which is processed after each phase, giving microtasks a higher priority.
Real-World Analogy: The Restaurant Model
Imagine a restaurant with a single chef (the main thread). Instead of waiting on each order to cook before taking the next, the chef delegates tasks like cooking or delivery to kitchen staff (the system/kernel). As each dish is ready, the chef serves it immediately. That’s the Event Loop in action: lightweight, efficient delegation and rapid turnaround.
Best Practices for Leveraging the Event Loop
To make the most out of the Event Loop:
- Avoid blocking the main thread with synchronous code like
fs.readFileSync()
or CPU-intensive tasks. - Use asynchronous APIs wherever possible.
- Offload heavy computations to worker threads or external services.
- Profile and monitor your Event Loop lag using tools like
clinic.js
or Node.js’sperf_hooks
.
By adopting these best practices, you can unlock the true power of Node.js’s event-driven nature.
Common Pitfalls to Watch Out For
Even though the Event Loop is powerful, it’s not magic. Here are a few things to be cautious of:
- Blocking the thread: Functions that take too long to execute will block the loop and delay all other operations.
- Excessive recursion or long loops: These can cause stack overflows or freeze the Event Loop.
- Neglecting microtasks: Overusing
process.nextTick()
or Promises can starve I/O callbacks.
Understanding the inner workings of the Event Loop helps prevent these mistakes and improves application performance.
Tools to Monitor and Debug Event Loop Behaviour
Want to see how your Event Loop is performing? Try these tools:
node --trace-events
: Offers detailed logs on Event Loop activity.clinic.js
: A Node.js performance profiling suite.perf_hooks
: Native module to track performance timings.
These tools offer deep visibility and can help identify performance bottlenecks or inefficient code paths.
Conclusion: The Engine Behind Node.js Performance
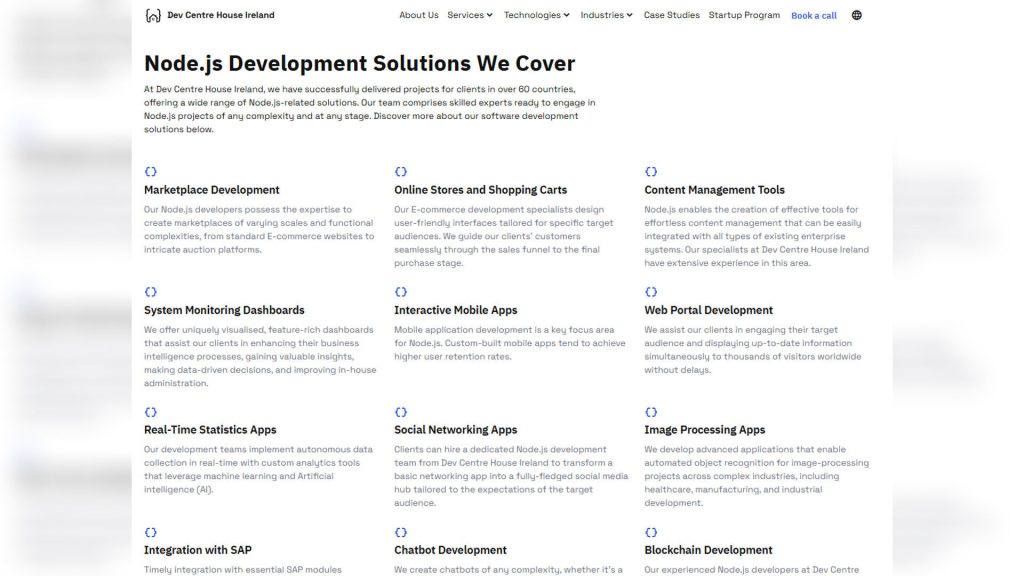
The Node.js Event Loop is the unsung hero of asynchronous JavaScript. It enables developers to write non-blocking, highly scalable applications using a single thread, which is especially crucial for building modern real-time web applications.
By grasping how the Event Loop functions and following best practices, you can unlock the full performance potential of Node.js. So the next time you’re handling I/O or writing a service that scales, remember it’s the Event Loop doing the heavy lifting behind the scenes.
Want to go deeper? Visit Node.js documentation or explore DevCentreHouseIreland’s Node.js overview to see how this runtime powers modern back-end development.